After introducing model()
for signal-based 2-way bindings, let’s dive into viewChild()
and contentChild()
for signal-based queries.
In case you’re not familiar with Angular queries, they existed before Angular 17.2 as the @ViewChild
and @ContentChild
decorators.
ViewChild is a way to get a reference on an HTML within the current component’s template. For instance, if we need our Typescript to access the instance of a given child component, I can use @ViewChild
like so:
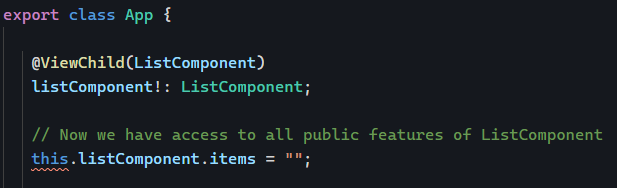
The new syntax gives us the same feature as a Signal:
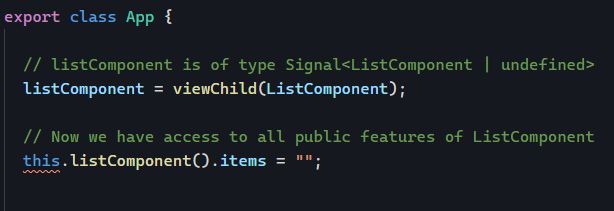
The same goes with ContentChild. The difference between ViewChild and ContentChild is that ViewChild looks for an element inside the component’s template, whereas ContentChild looks for an element projected by the parent component into the ng-content
element of the current component using content projection. You can learn more about content projection with this tutorial:
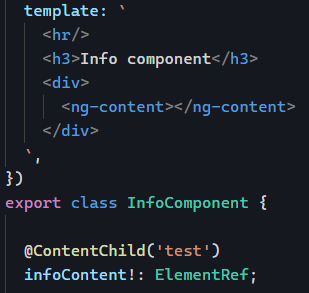
The above code will look for an element with a template reference variable called test in the projected ng-content
.
We can achieve the same feature with the new contentChild()
function, which returns a Signal:

Differences between these new functions and the old decorators
These new functions bring a few extra interesting features. For instance, we can get rid of possibly undefined
values by making a query required
:

Decorator-based queries were satisfied once the component was fully initialized, which means that running side effects required the use of specific lifecycle methods:
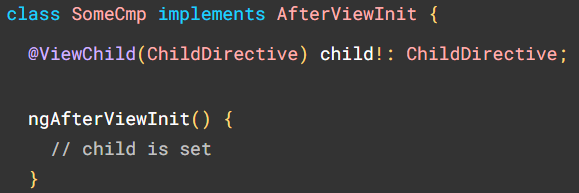
With signal-based queries, we receive a signal so we can rely on computed()
or effect()
to run such side-effects:

This approach is less intrusive and requires knowing just one thing: Signals! No more lifecycle methods are needed. You can find these different examples in action on Stackblitz here.