Angular 17.2 brought more features to the signal-based components approach, where almost everything in our components will become signals. We’ve already covered that inputs can be signals since Angular 17.1.
As an Angular developer, you’ve probably used ngModel
in the past to create two-way data bindings. You might have created your own 2-way bindings using the approach covered in this tutorial, too.
All of that becomes even easier with Angular 17.2, as we can now do the following using the model
function:

The above syntax means that we can pass a value to NameEntryComponent
‘s age
property as follows:

We can even have a 2-way binding (meaning something that acts as both an input and an output) by using the “banana in a box” syntax as follows:

This gets even better as we can specify that a value is required for that model input using model.required
:
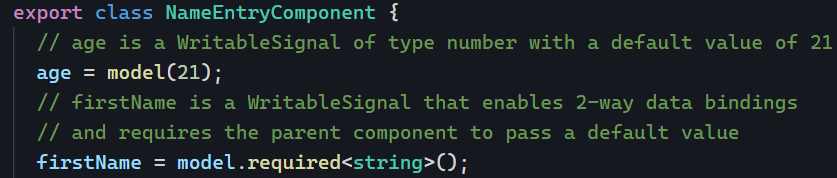
Since age
and firstName
are signals, we can update their values using set
or update
:
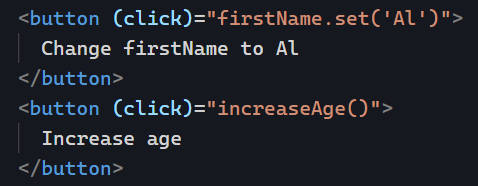
Where increaseAge
is a method that uses an arrow function (as those aren’t supported in Angular templates):
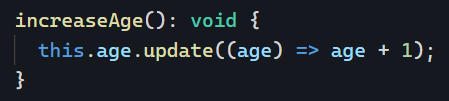
You can see this example in action on Stackblitz here.
We can pass data to models using regular component properties:
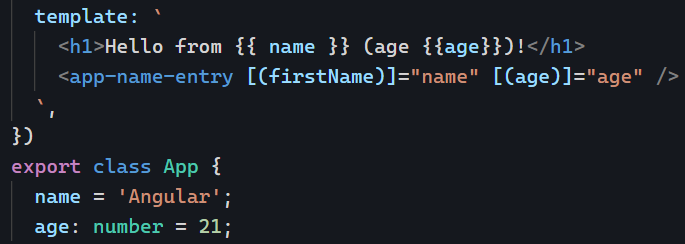
But it gets even better as we can also use signals as-is with these 2-way bindings:
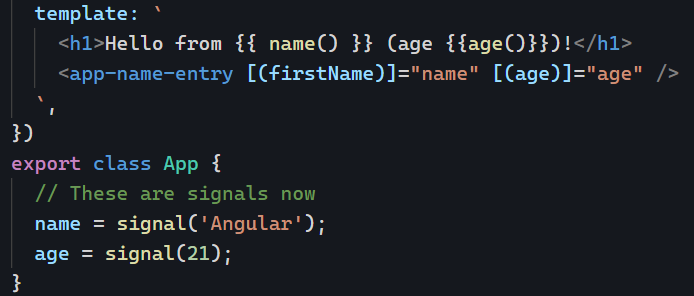
You can see this new example in action on Stackblitz here. Next week, I will cover 4 more functions available since Angular 17.2, all signal-based as well!