We introduced RxJs Subjects a few weeks back.
BehaviorSubject
The most common type of Subject used with Angular is BehaviorSubject
. Why is that? Because a BehaviorSubject
has two exciting features that a plain Subject
does not have:
- It starts with a default value, which means it is never empty.
- When we subscribe to a behavior subject, it will immediately give us the last emitted value.
Imagine subscribing to a magazine and receiving its latest published issue immediately. That’s what a BehaviorSubject
does. This is helpful if you have components that need to know about the app’s current user. When the component subscribes to the “current user,” we want to get that info immediately and not wait for the next user update.
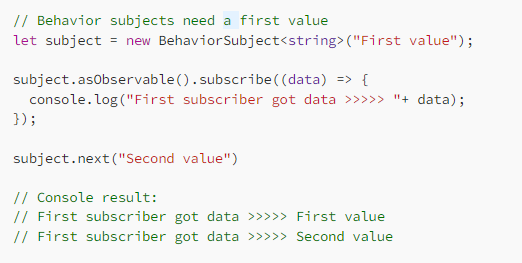
Also, since behavior subjects always have a value, they have a getValue(
) method that synchronously returns the current value.
ReplaySubject
A ReplaySubject
is very similar to a BehaviorSubject
, with two key differences:
- No default value
- Can replay more than just the last value
The constructor parameter determines how many values should be replayed to new subscribers:
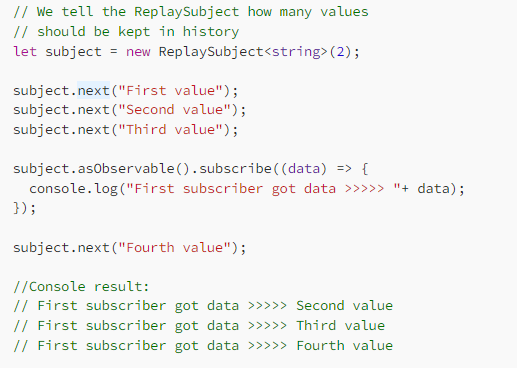
Subject
A plain Subject
has none of the above capabilities. When a value is emitted, current subscribers receive it, but future subscribers won’t. There is no replaying of the latest value(s), which makes plain Subjects less interesting to work with.