Another Angular 17 update is the release of a new DevTools panel called Injector Tree. It’s a good option to visualize both your element hierarchy and injector hierarchy, and it is accessible as a third tab in the Angular DevTools browser extension available for Chrome, Firefox, and Edge:
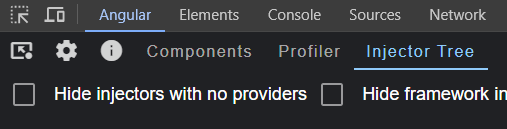
When we click on that tab, a tree view of all hierarchies shows up on the screen:
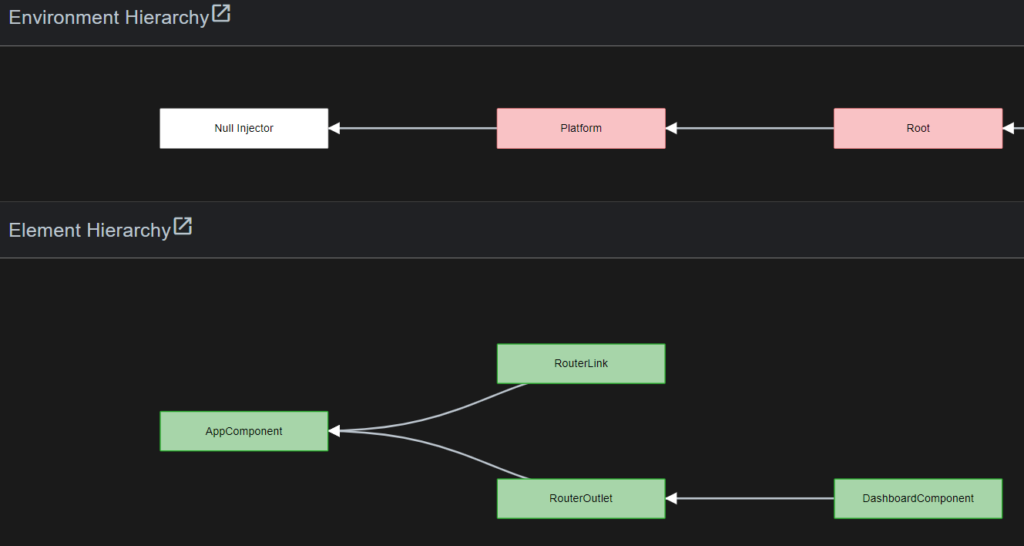
For a clearer view, we can check the option “hide framework injectors” to focus on our code:
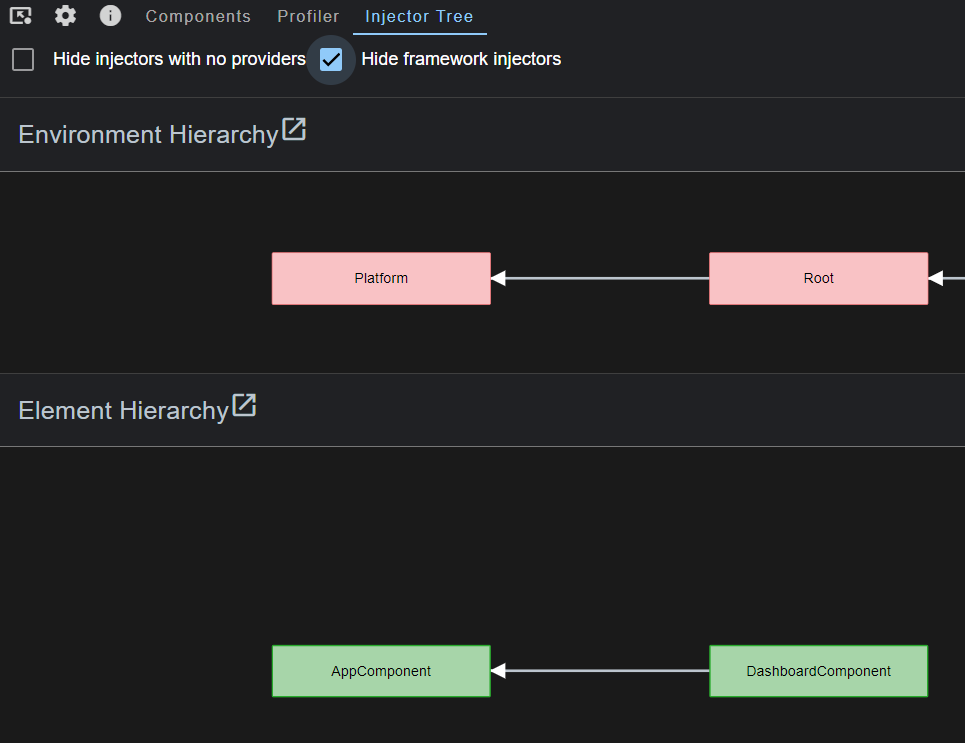
Clicking on an injector (such as root) shows the list of all injectables/services available in that injector:
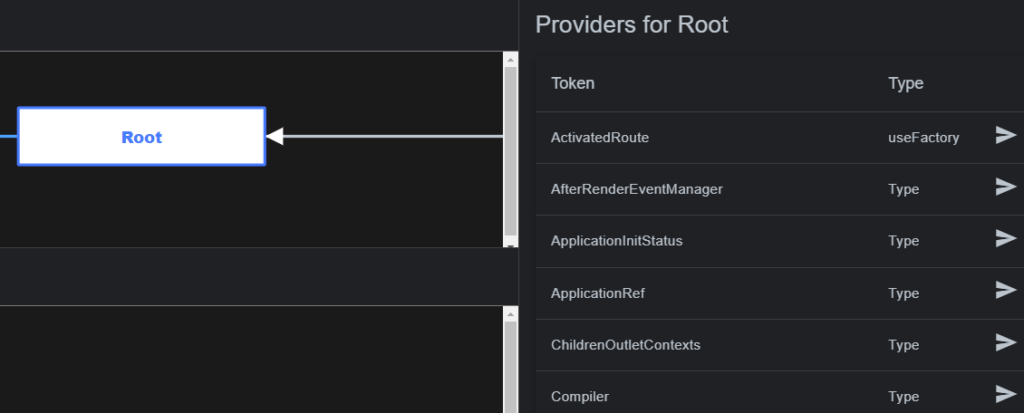
This new tab seems to be just getting started, and more information will be added later. Even though the Devtools is a browser extension, the Injector tree tab only works for apps using Angular v17+. I tried an Angular v16 app with no success.