Yesterday, we touched on how to change the locale of our Angular applications. The next step is to fully internationalize our apps by translating all text into different languages.
While Angular has its localization implementation to internationalize applications, the most popular library used for such a task is ngx-translate.
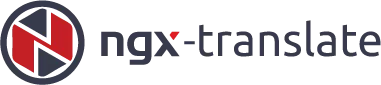
Why not use Angular’s internal implementation? The main reason is that Angular builds one version of your application per locale. As a result, if you have to support 20 different locales, your Angular build would output 20 different applications. This means that changing the site would result in a complete reload of the application. This also means that changing a translation requires a complete rebuild and redeploy of the application.
ngx-translate is much more flexible because it relies on pipes, directives, and services to translate our text at runtime instead of build-time. Here are some examples:
With TranslateService
:

With TranslatePipe
:

With TranslateDirective
:

Translations come from specific files where translation keys ("HELLO"
in my example) are mapped to actual values in each language. ngx-translate
supports different formats out of the box (such as JSON and PO).
Here is an example of English translations stored in en.json:
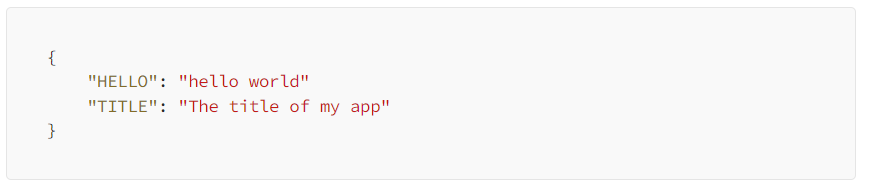
And the same translations in French in fr.json:
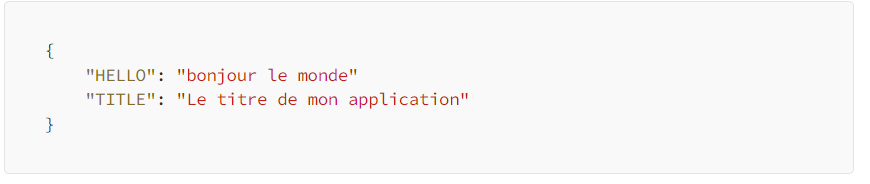
All we have to do is indicate where the translation files are located and ngx-translate
will download them as needed based on the locale.
Defining which languages are supported as well as which one to use by default can all be done with the TranslateService
from the library:
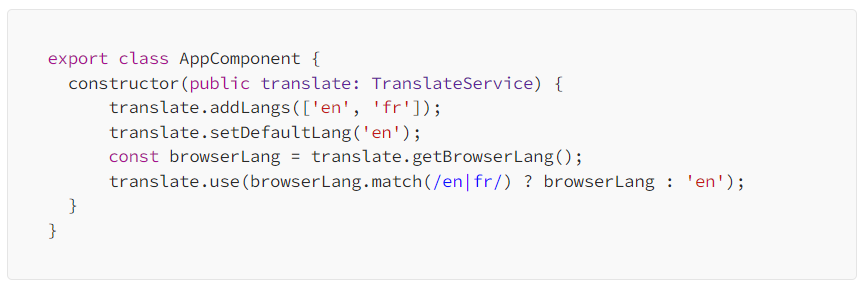
You can see a live example of ngx-translate
in Stackblitz here. If you want to try it, you can use my ngx-translate step-by-step tutorial.