Let’s get back to our series on state management with this post. So far, we covered that state management libraries give us a global State that gets updated using Actions and Reducers. I haven’t told you yet that typical Redux Reducer functions are meant to be synchronous. In other words, you’re not supposed to make an HTTP request or anything asynchronous in a reducer.
So, how do we implement asynchronous tasks? This is known as a side-effect (because it involves a third party such as a server) or just Effect.
When we use Effects, the idea is to have an Action that triggers the Effect, and once the Effect completes, another Action is triggered:
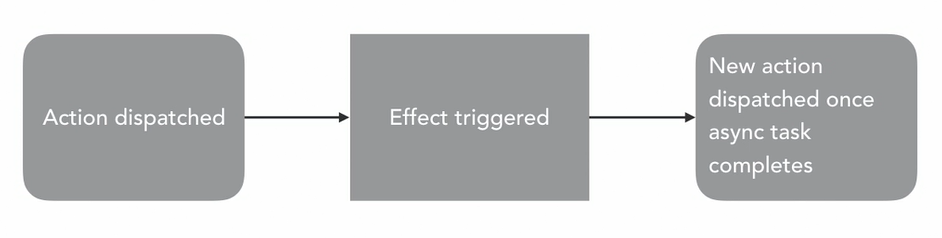
For instance, in our current switcher example, we could have an Action called LOAD_EXCHANGE_RATES
. This Action would trigger an Effect to make an HTTP request and fetch exchange rates from an API. For instance, the original Action would also update the State to make it a “loading” state.
Then, once the HTTP request completes, the Effect would dispatch another action, EXCHANGE_RATES_LOADED
and pass the data as its payload so it gets stored in the State.
Effects are implemented differently based on the state management library
- NgRx
NgRx effects rely heavily on RxJs and look like this:
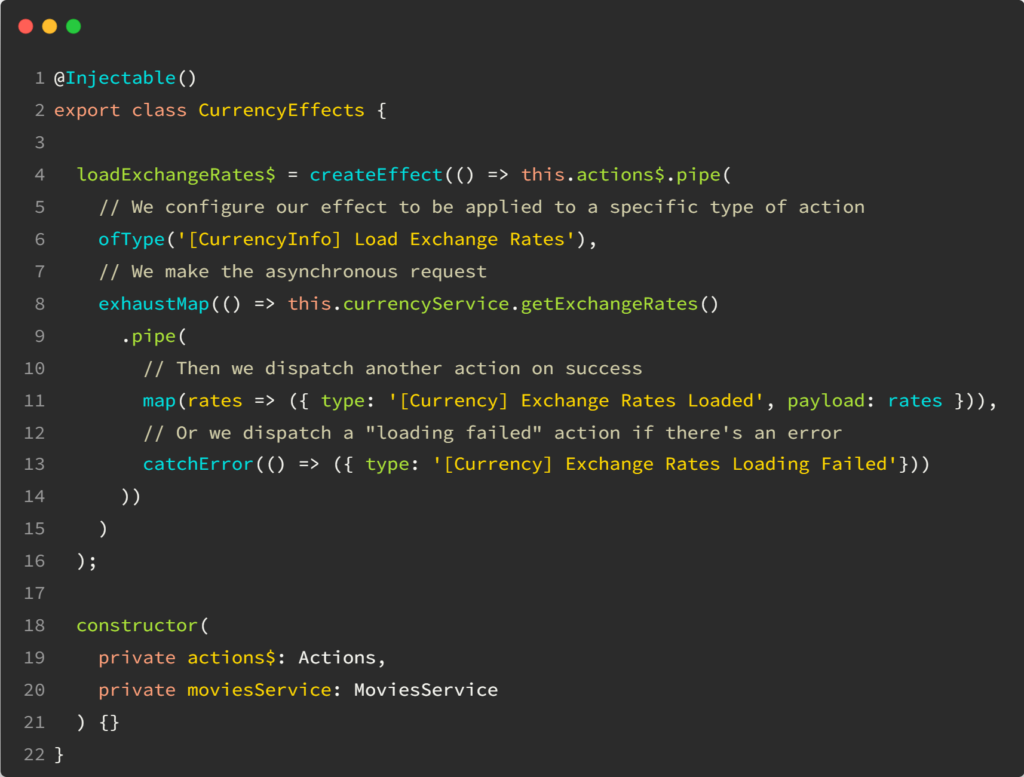
As you can see, the Effect code is verbose, but every single step makes sense: We turn an action into an asynchronous request that dispatches other Actions upon success or failure.
If you want to learn more about NgRx, I have this video course on LinkedIn Learning.
2. NgXs
NgXs has a different approach and doesn’t have effects. Instead, all actions have four states by default:
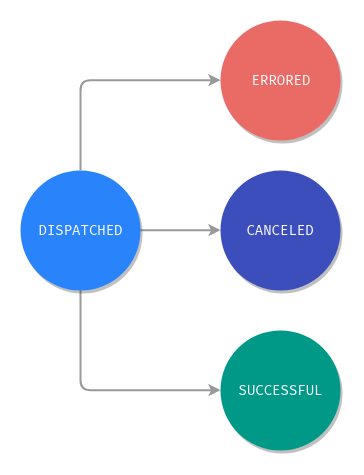
As a result, action handlers (reducers) can use asynchronous requests without needing any effects:
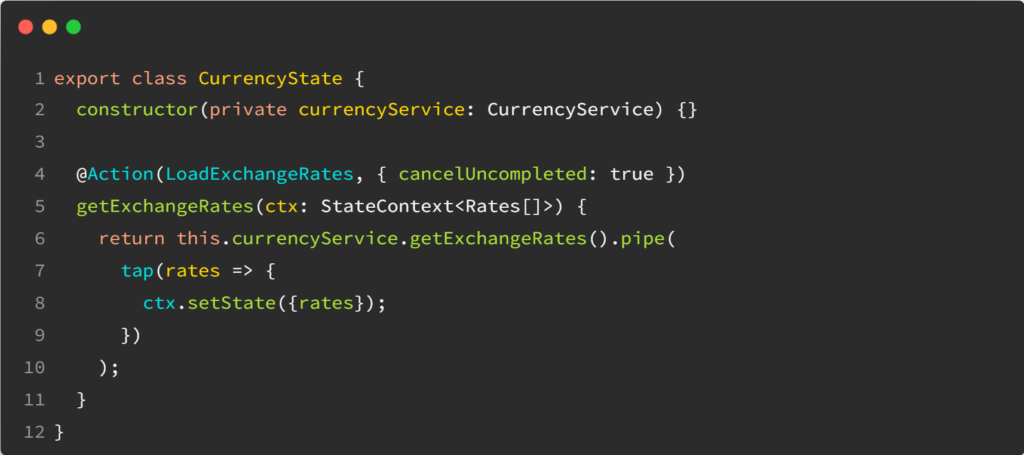
As you can see, even if the Redux concepts remain the same, every state management library has a different approach. I like NgXs because of its simplicity. The code is more to the point and less reliant on RxJs operators.