In the past few weeks, we’ve talked about how to cache data with HTTP interceptors, with Subjects, and with shareReplay
.
While all these options work, they are intrusive and need additional code for your application. Another option doesn’t require touching your application code; it only requires additional configuration, and that’s using a service worker.
What’s a service worker?
A service worker is a small piece of Javascript code that runs in the browser independently from your application. A service worker can be an HTTP proxy between your front-end code and server. It can intercept all HTTP traffic between your Angular application and any remote server.
How to enable service workers in Angular?
Run the command: ng add @angular/pwa
That’s it! You can read more about what the command does in the official documentation. The idea is that instead of writing our service worker code, we let the Angular API take care of that and generate the service worker based on our config.
How to configure what to cache, and for how long?
Angular generates a file called ngsw-config.json
. In that file, you can specify which URLs you want to cache, for how long, and if you wish to optimize for freshness (use the cache only if the application is offline) or performance (use the cache as much as possible even if the app is online).
For instance, data that doesn’t change very often (a list of countries or currencies) could be cached for weeks or months, while dynamic data (a list of Tweets or user preferences) could be cached for just a few minutes:
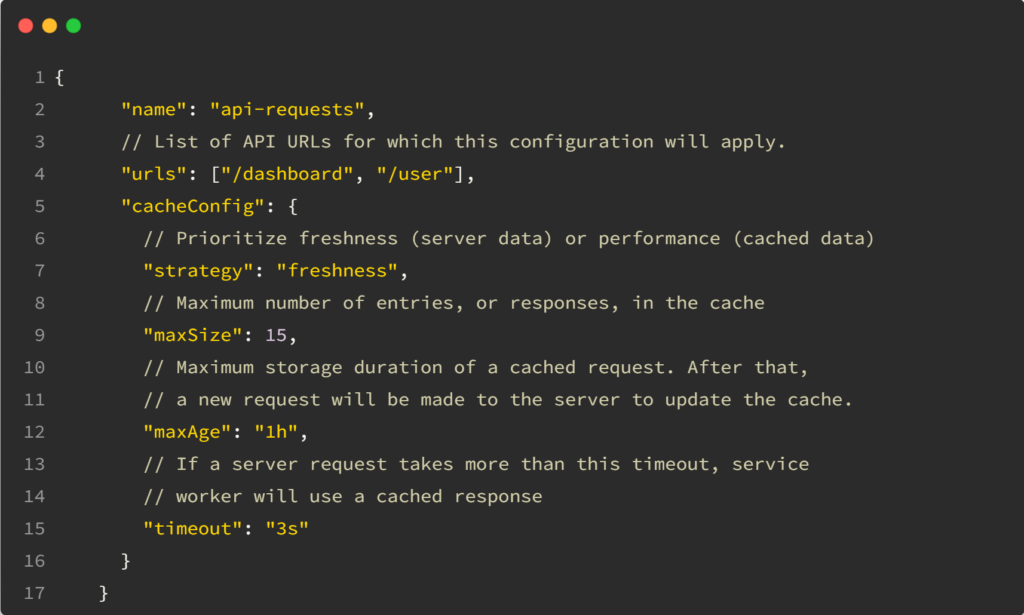
You can find a complete example of such ngsw-config.json
here. The full documentation for all supported options can be found here.
Service workers are part of Progressive Web Apps (PWAs). If you want to learn more about that, here’s a quick PWA tutorial of mine.