Before I dive into the solution of code challenge #3, I wanted to mention that my next FREE workshop is scheduled for April 4th. It will be all about getting into RxJs from scratch, and you can register here. I’ll start from the basics and move on to more advanced topics, such as subjects and operators, and you’ll be able to ask me questions as we go through the content and exercises together.
As always, it’s a free workshop, but your coffee donations are always welcome.
Let’s get into our code challenge solution. The goal was to “connect” two different dropdowns so that the selection of the first dropdown (a continent) would update the contents of the second dropdown (a list of countries for that continent):
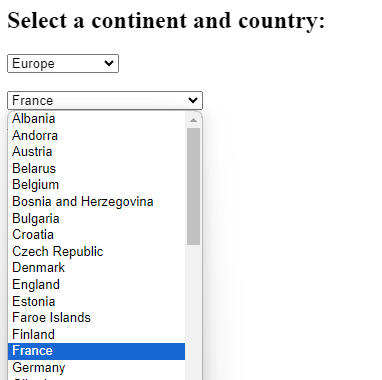
The challenge is that countries depend on two different Observable data sources:
- An HTTP request to an API to get the list of all countries in the world
- The currently selected continent (a reactive form
select
dropdown)
In other words, we start with the following code:
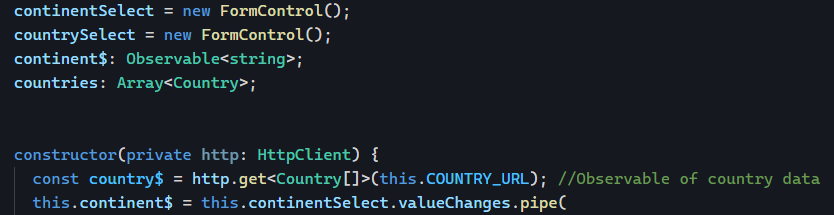
Our goal is to combine data from both Observables into one result. Ideally, such a result would be updated whenever any of the Observable sources are updated (e.g., a new continent is selected by the user, or a new list of countries is available from the API).
As a result, we can use the withLatestFrom operator to make that “connection” between our two Observables:

Such an operator returns an array of all the latest values of our source Observables in the order in which they were declared. As a result, we can use the map
operator to receive that array and turn it into something different:

Inside that operator, we decide to return a new array. The continent remains the same, and we filter the list of countries based on that continent:

FInally, we can use tap
to run some side effects. In our case, we update the list of countries used by the dropdown, and we set the country dropdown value to the first country of that list to ensure that a country from a previous continent doesn’t remain selected:

And that’s it! You can see that code in action on Stackblitz here.
A few of you sent me their own solutions to the challenge, and a recurring theme was that most of you didn’t “connect” the Observables and just assumed that the list of countries would already be downloaded and available by the time the user selected a continent. Something along those lines, where this.countries
would be received from the API before that code runs:
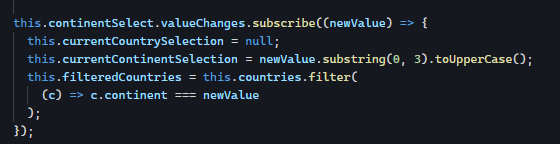
While the above code would work most of the time, if a user selects a continent before this.countries
is defined, an error would be thrown and the corresponding subscription destroyed, which means the above code would not run anymore when the user selects a new value. As a result, using operators is safer.
Another trick in my solution is that I didn’t use any .subscribe
in my code, which means I don’t have to unsubscribe, as I let the async
pipe do so for me automatically:
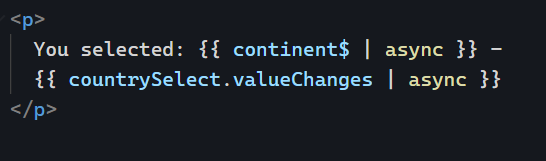
That’s it for code challenge #3! Next week, I’ll send you updates from ng-conf in Salt Lake City. If you’re around, feel ree to come say hi!