As mentioned earlier in this newsletter, the size of your Javascript matters a lot, as our code has to be downloaded first, then parsed and interpreted by a browser, which will get slower and slower as the size of your app increases. This is why we want optimized production builds. And this is also why it’s always a good idea to keep track of the size of your production code after each build.
Fortunately, the Angular team has our back and integrated build budgets in the Angular CLI. You can use those budget settings to decide when to get a warning or even fail a build it your code becomes too big. This configuration happens in angular.json
:
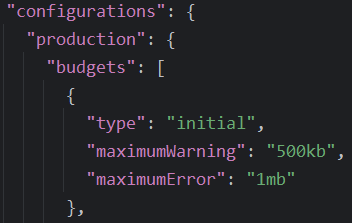
The above (default) budgets would warn you if your Javascript exceeds 500Kb in size and fail once the build exceeds 1Mb. Those are already part of your projects, so you don’t have to do anything to use them.
If you do continuous integration, your build would fail right after the commit that degraded your bundle size, making it easy to troubleshoot and fix the issue.
Most of the time, dependencies are the culprit. I remember coaching a client that needed some Excel-like features in the browser, and their build exploded to over 25MB because of a massive monolithic Javascript Excel library… Thanks to the build error, we knew that this library wouldn’t work so we chose a lighter one instead.
In the past, I’ve also inherited projects where I would track our build size version after version. My client was amazed to see that despite adding features, the build was getting smaller and smaller after each release, thanks to Angular always being better at tree-shaking and having the incentive to clean up old code and make it smaller. When you start tracking a metric, you want to improve it!