In the past weeks, we covered a few different RxJs operators in this newsletter. RxJs is the most difficult thing to learn about Angular, and that’s because the technology is a mix of concepts that are not always obvious on their own: asynchronous data and callbacks, functional programming, streams, etc.
My favorite resource to learn more about RxJs is to use a website called rxmarbles.com. Why? Because that website features interactive diagrams of RxJs operators.
Let’s take an example with mergeMap
. Here’s the official definition of mergeMap
:
Projects each source value to an Observable which is merged in the output Observable.
Rxjs.dev documentation
And here’s an interactive diagram from RxJs marbles for mergeMap
– I just recorded my screen while interacting with the diagram by dragging data around:
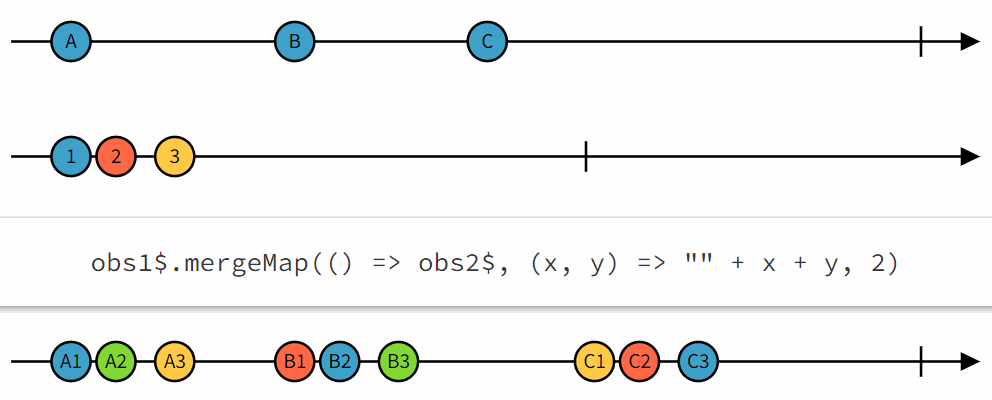
They say a picture is worth a thousand words. An interactive picture is even better, and that’s exactly what rxmarbles.com is. No text, just interactive diagrams.
Note: Not all operators are documented on that website, just some of the most useful ones. At times, the operator’s signature or name is slightly different depending on the version of RxJs you’re using. Still, overall, RxJS Marbles remains one of the very best tools for understanding operators.