Who doesn’t like faster builds? Since Angular 2, the Angular team has consistently worked on making our builds quicker and faster. Angular 16 included a developer preview of a new build system based on esbuild and Vite.
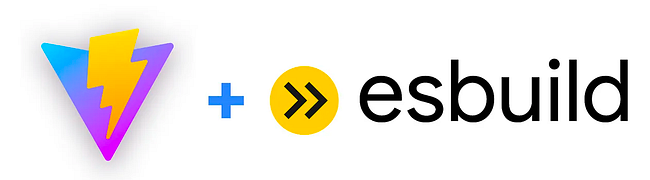
With Angular 17, this feature is now enabled by default for new apps, with reported speed improvements of over 67% in most applications.
To enable this new build system in existing apps (that use v16+), open your angular.json
file and look for all the places where @angular-devkit/build-angular:browser
is used:
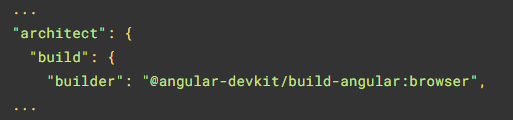
Then, replace those instances with @angular-devkit/build-angular:browser-esbuild
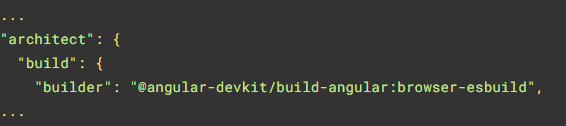
And your next run of ng serve
or ng build
will automatically use this new build system. Nice and easy! If you use server-side rendering or have tweaked the builders in the past, you’ll want to look at the extra instructions listed on this page.