Let’s say we want to trigger an action when the user hits enter on their keyboard. We could do something like this, capturing the keydown
event and then decide what to do based on the entered key:

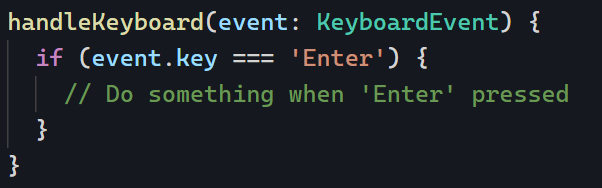
While the above works, it’s pretty verbose. Instead, we can make our binding more specific by doing the following:


Now, that’s a lot more specific and less verbose. The same goes for class bindings. Here’s a lengthy example:

Since all we want to do is toggle the green class on or off based on a boolean expression, the following syntax is shorter and better:

You can see these examples in action on Stackblitz.